Example 1a: Basic Carousel
Each child element inside the <it-carousel>
element will be treated as a slide.
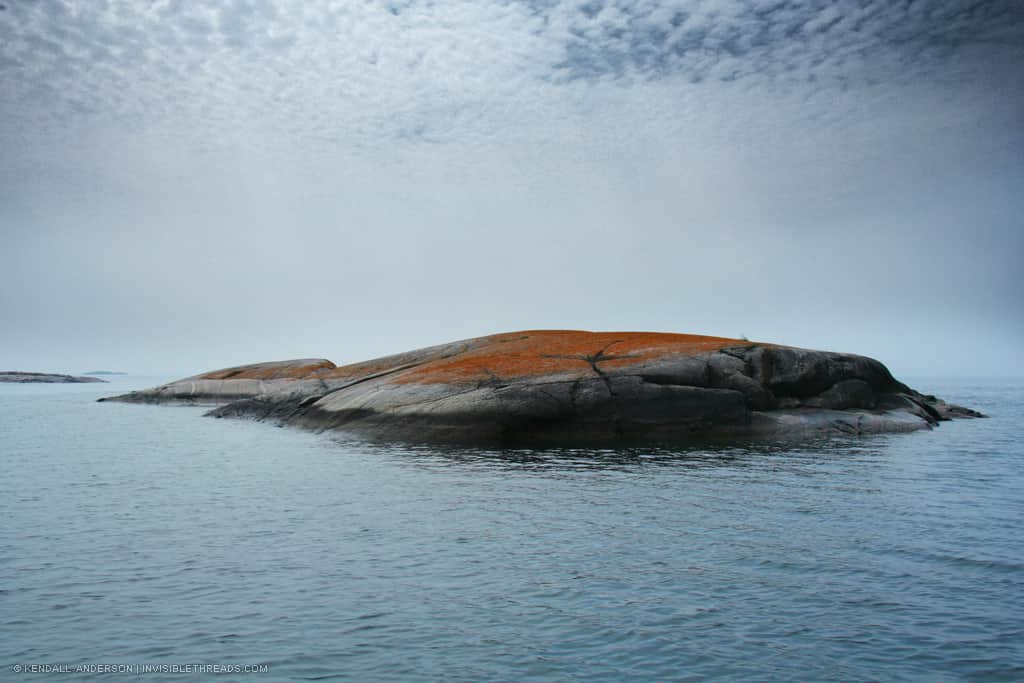
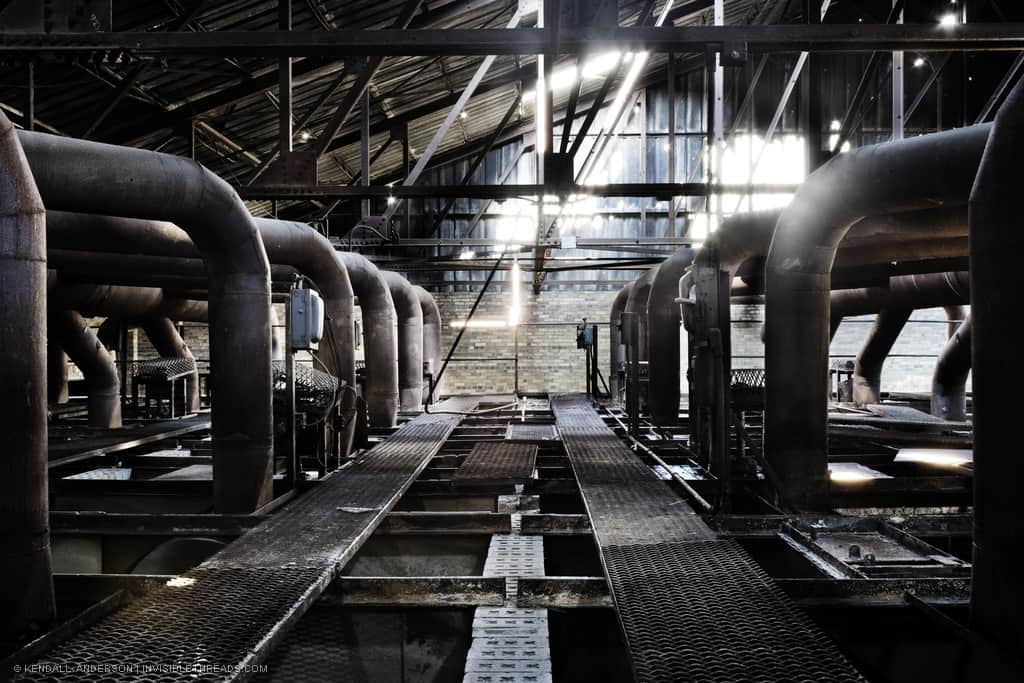
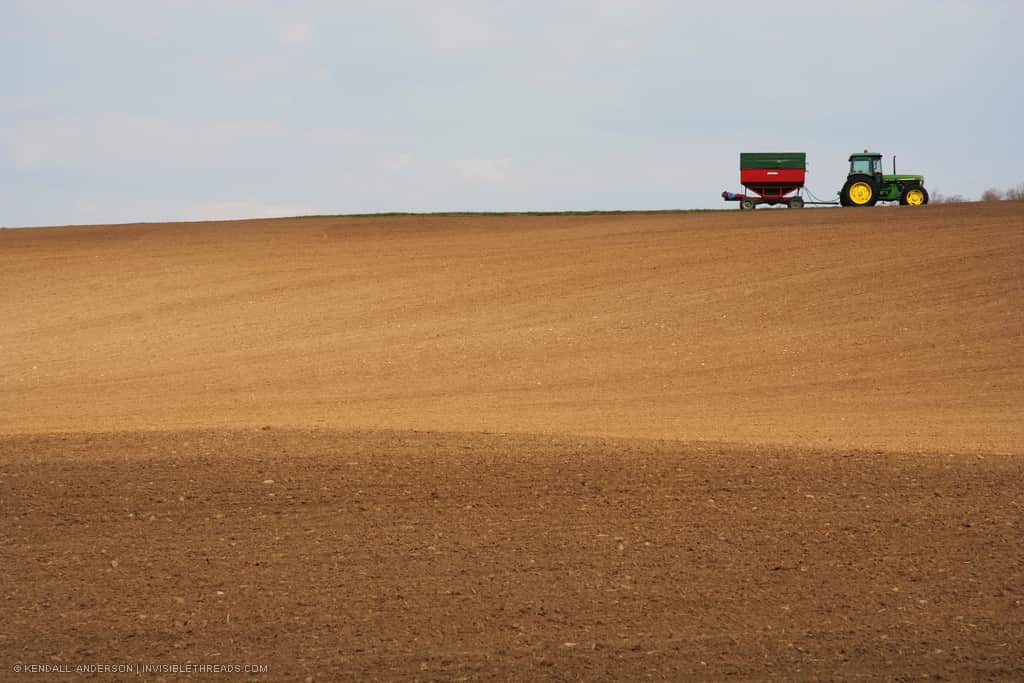
Example source
<it-carousel>
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 1b: Initial slide to display
By default, the first element in the carousel is shown first. You can change this by setting the initial-slide
attribute to the index of the slide you want to show first. A value of "1" is the first slide in the carousel.
In this example, show the 3rd item at first.
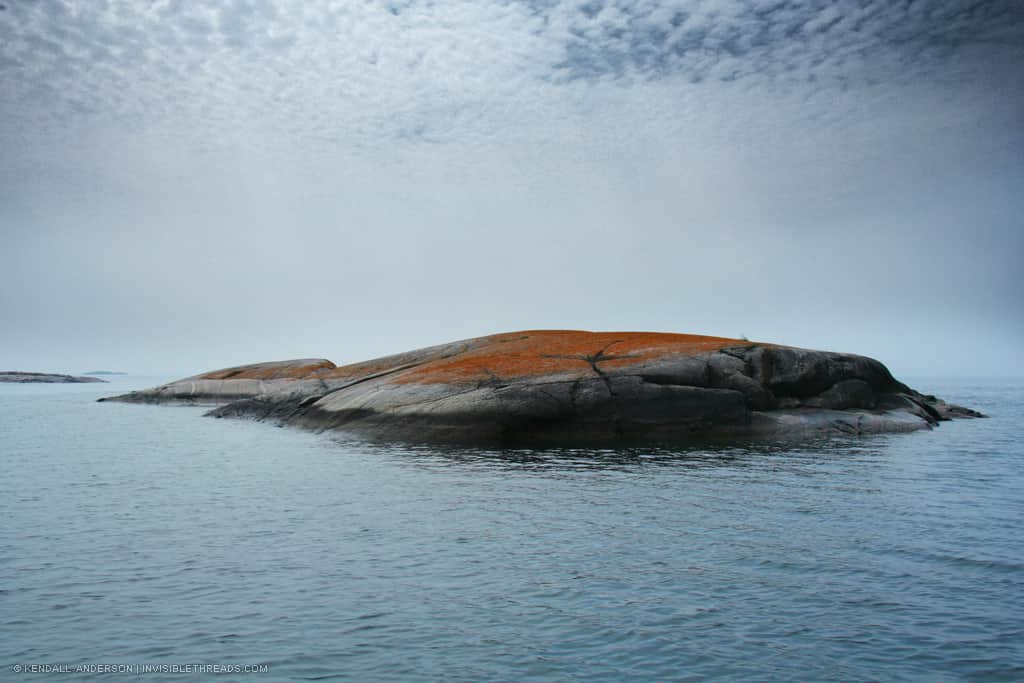
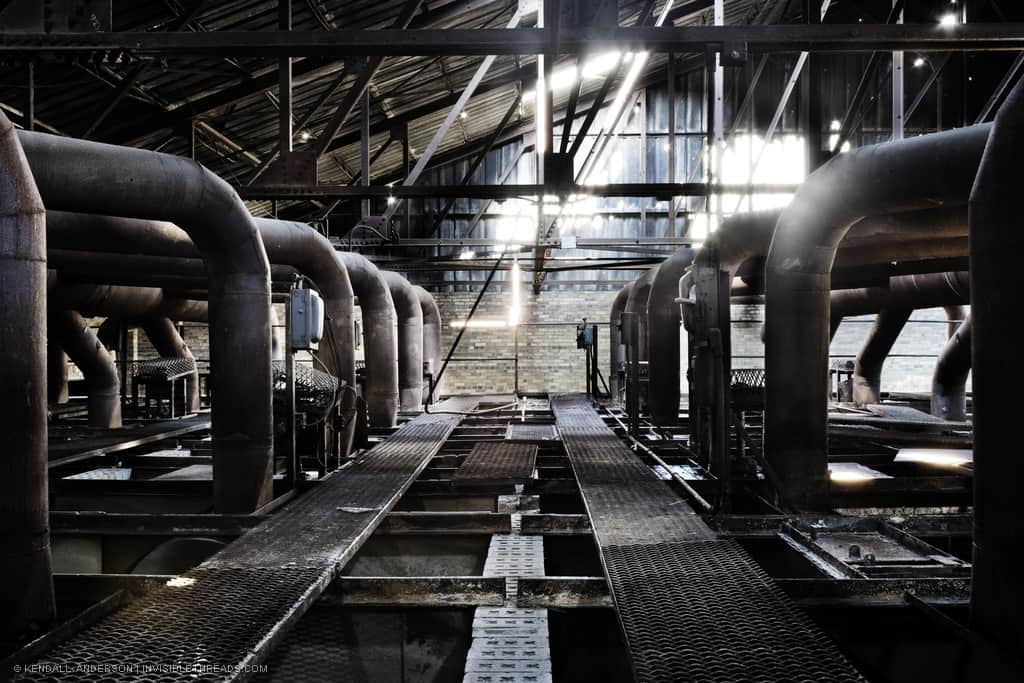
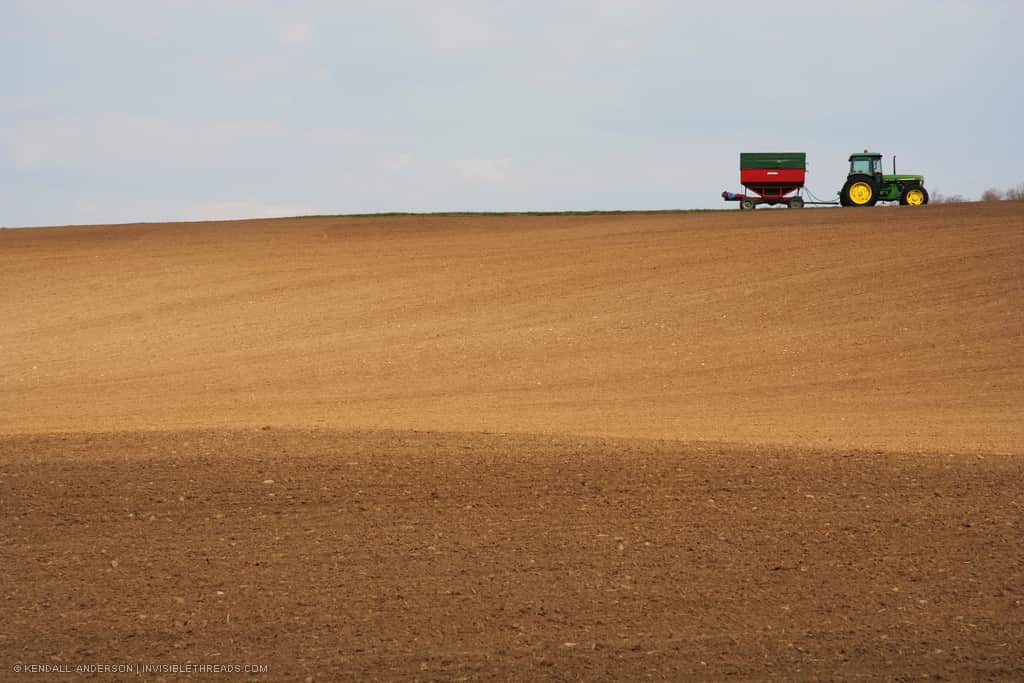
Example source
<it-carousel
initial-slide="3">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 2: Custom button labels
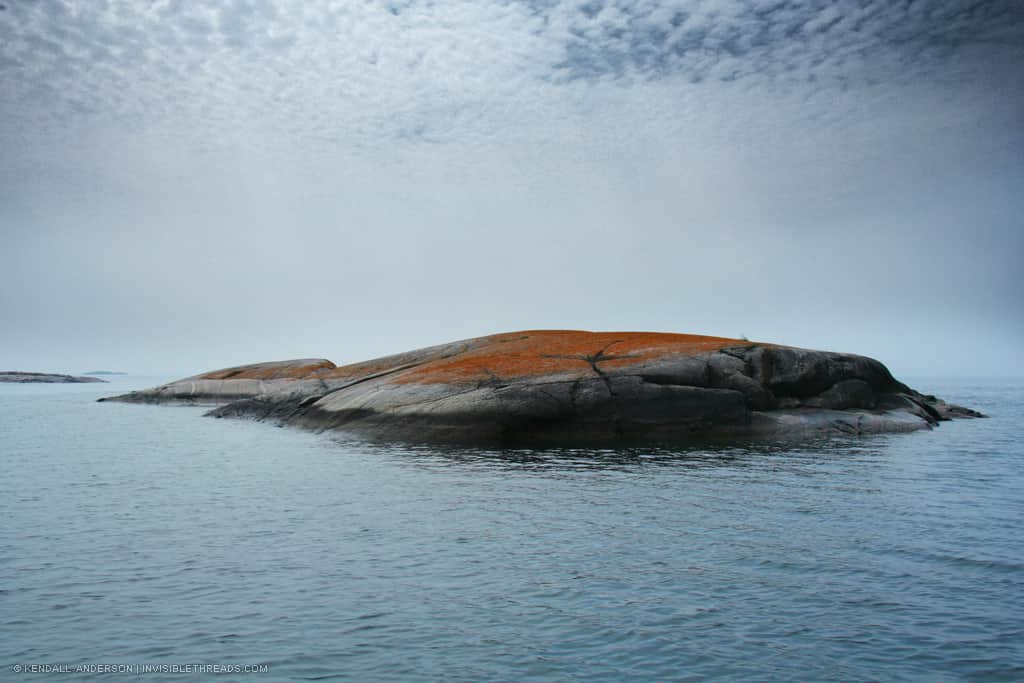
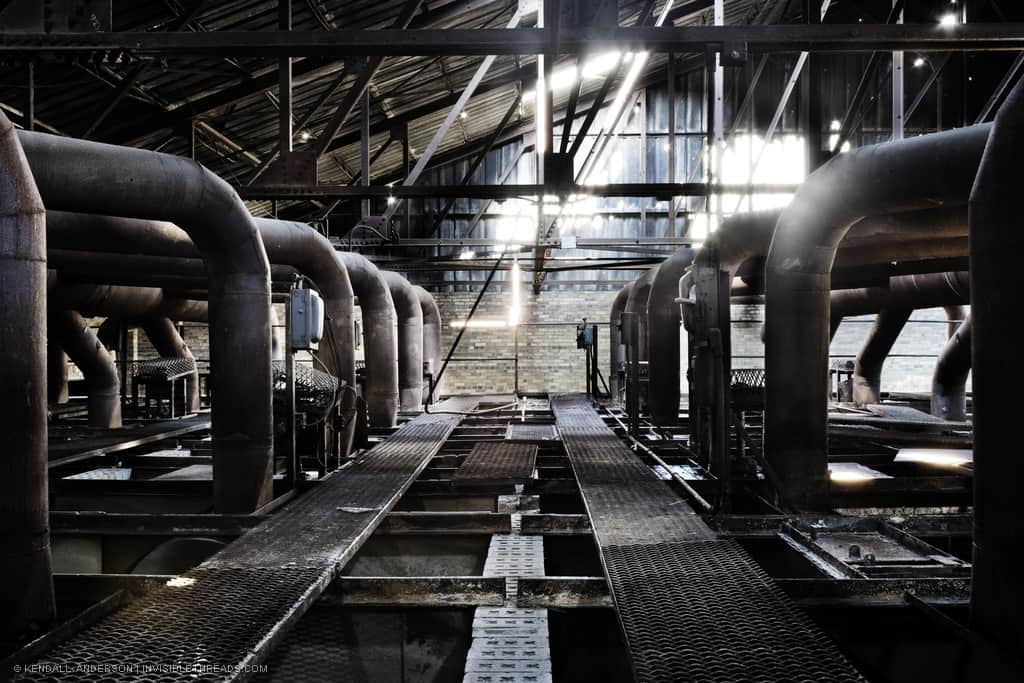
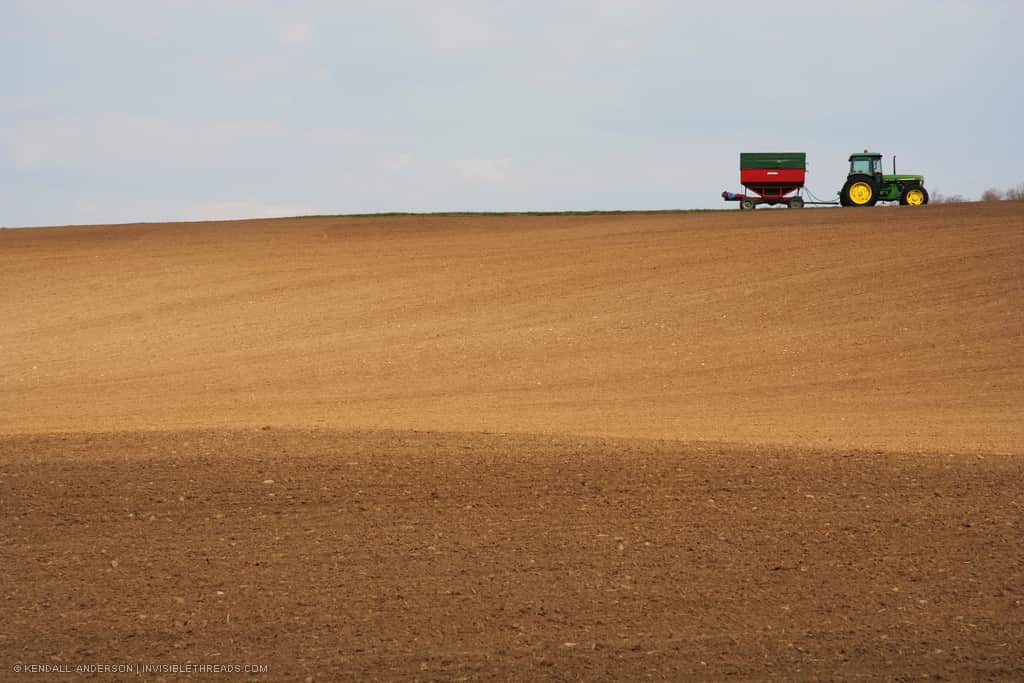
Example source
<it-carousel
label-prev="« Go back"
label-next="Go forward »">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 3: Custom button labels for screenreaders (ARIA labels)
(You'll only be able to see this by inspecting the live source code, or hear it by using a screen reader.)
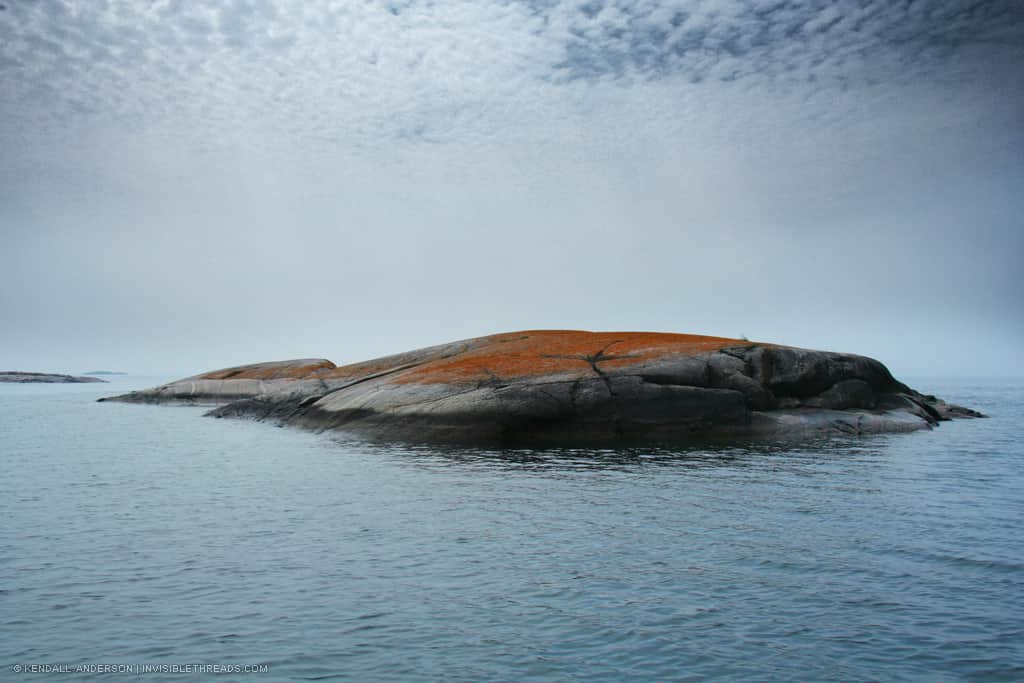
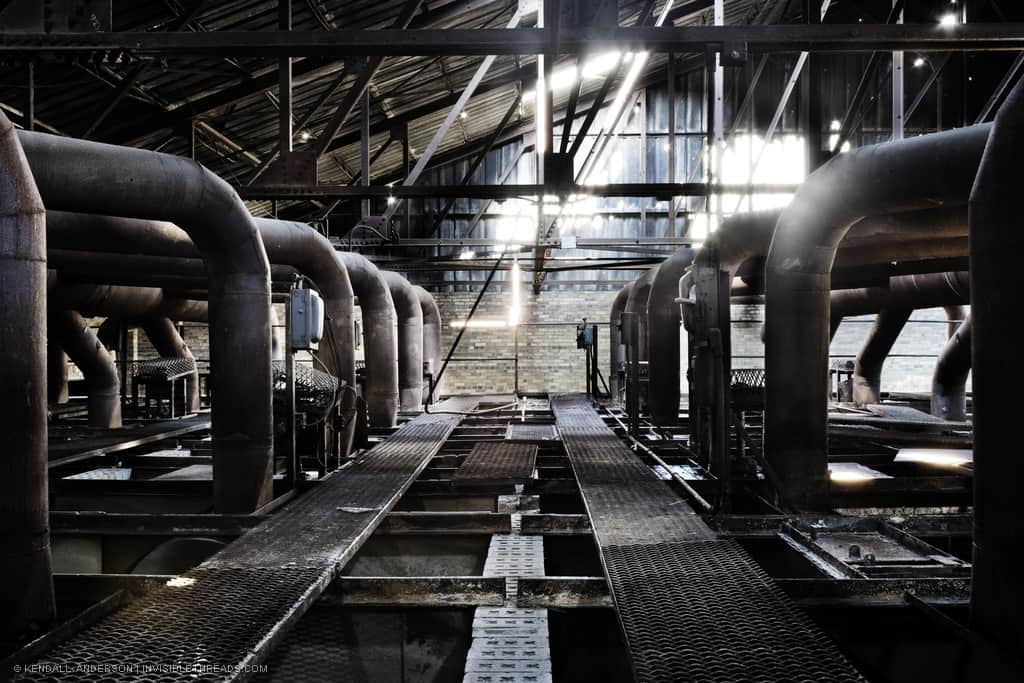
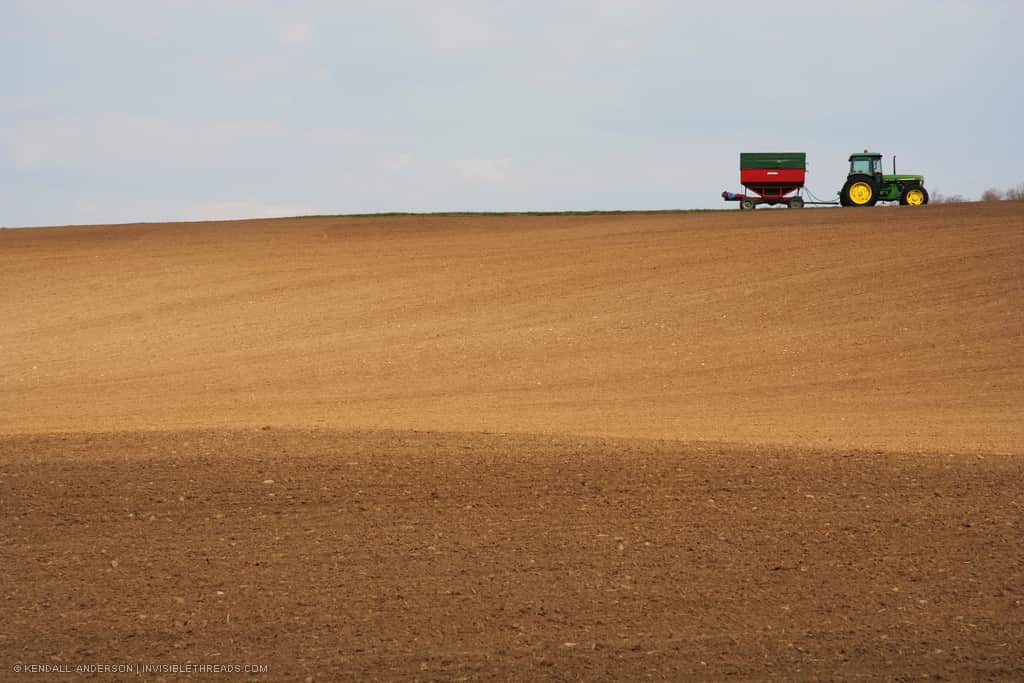
Example source
<it-carousel
aria-prev="Back message for screenreader users only"
aria-next="Next message for screenreader users only">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Important: It should be a rare case where you need to specify both a label **and** the ARIA label. Do NOT specify the aria-*
properties unless you fully understand accessibility and have a very good reason for the ARIA label being different than the visible <button>
label!
Example 4a: Pagination
Add the pagination
attribute to the <it-carousel> element to enable pagination.
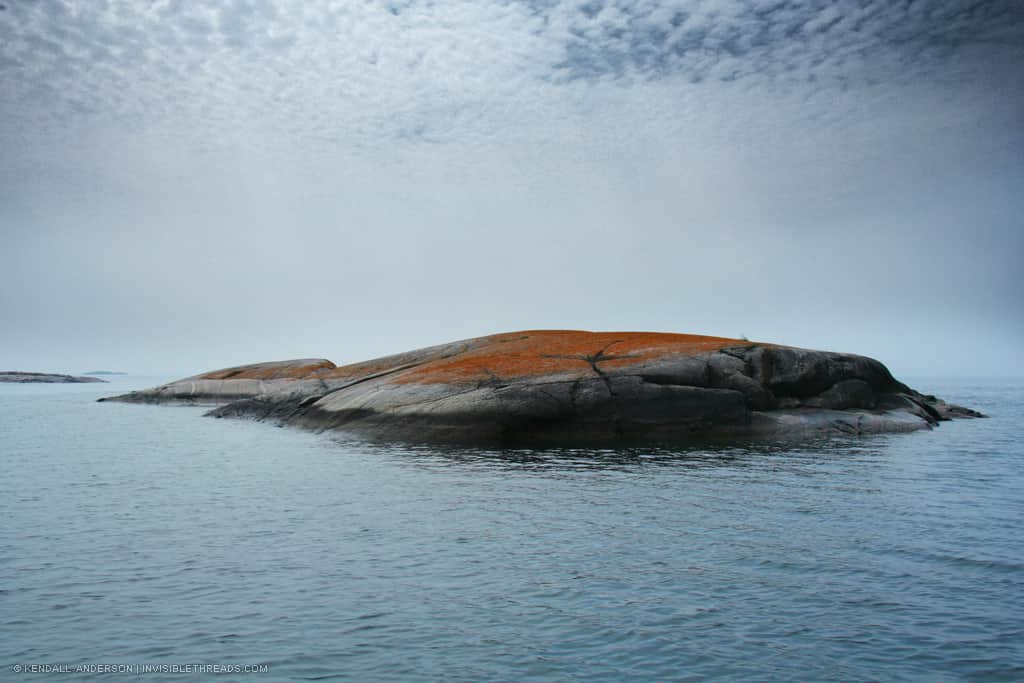
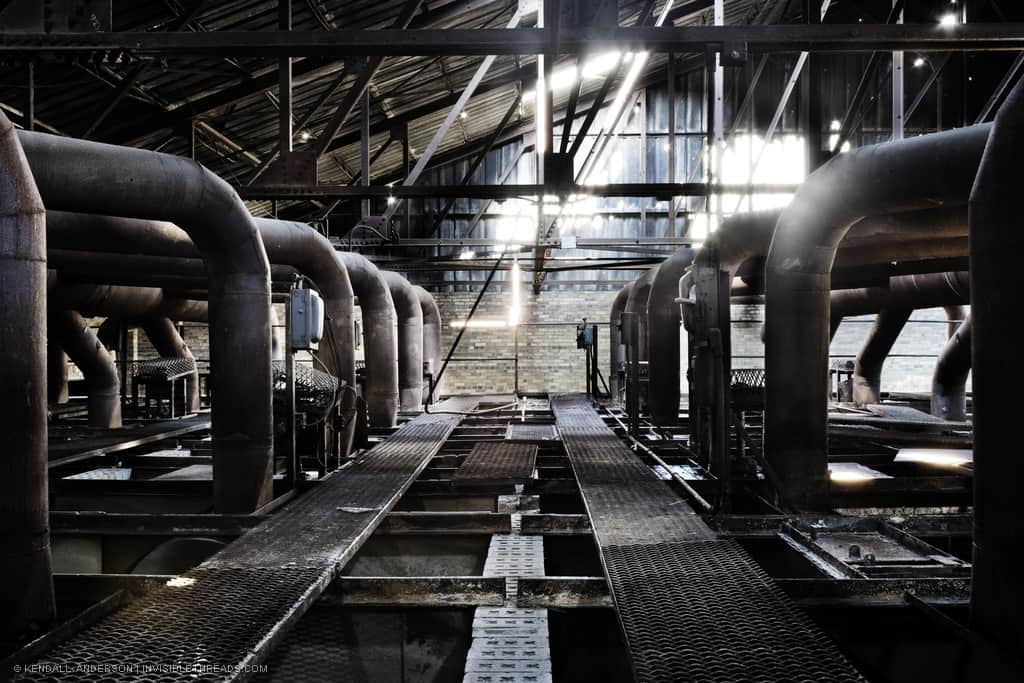
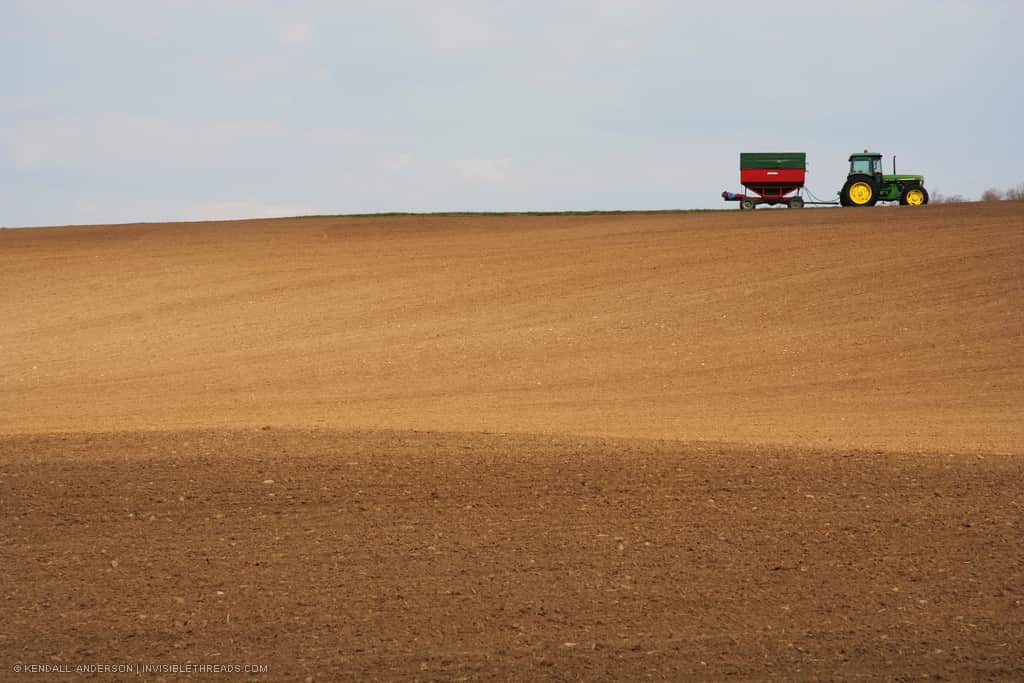
Example source
<it-carousel
pagination>
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 4b: Remove labels from pagination buttons
Set the pagination-label
attribute to an empty string to remove the labels inside the pagination buttons.
We'll also provide some custom styling so the buttons still appear.
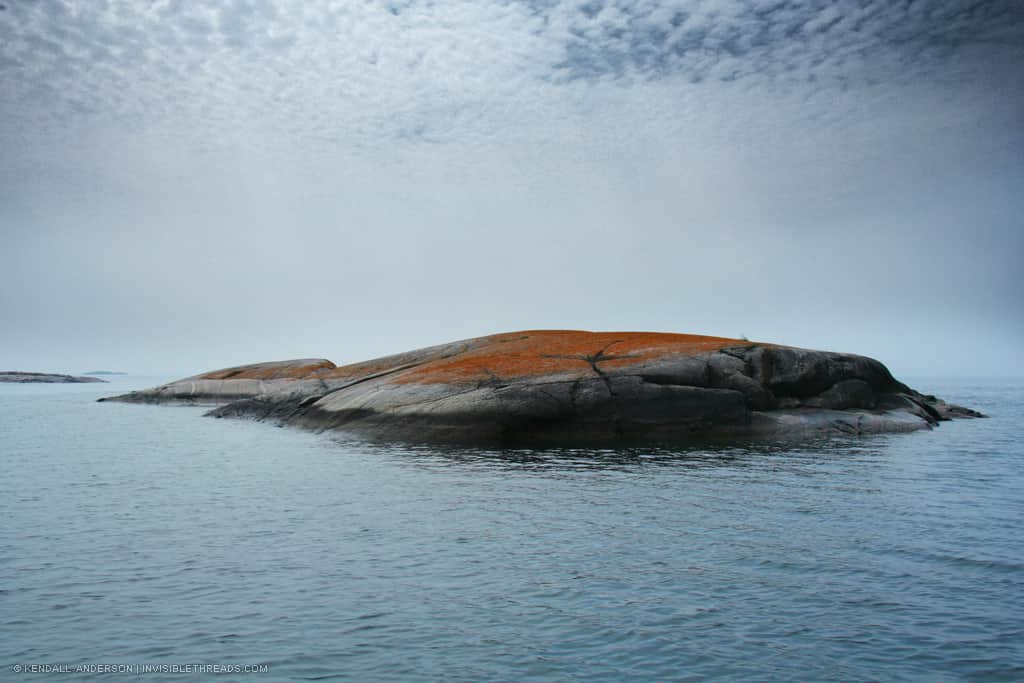
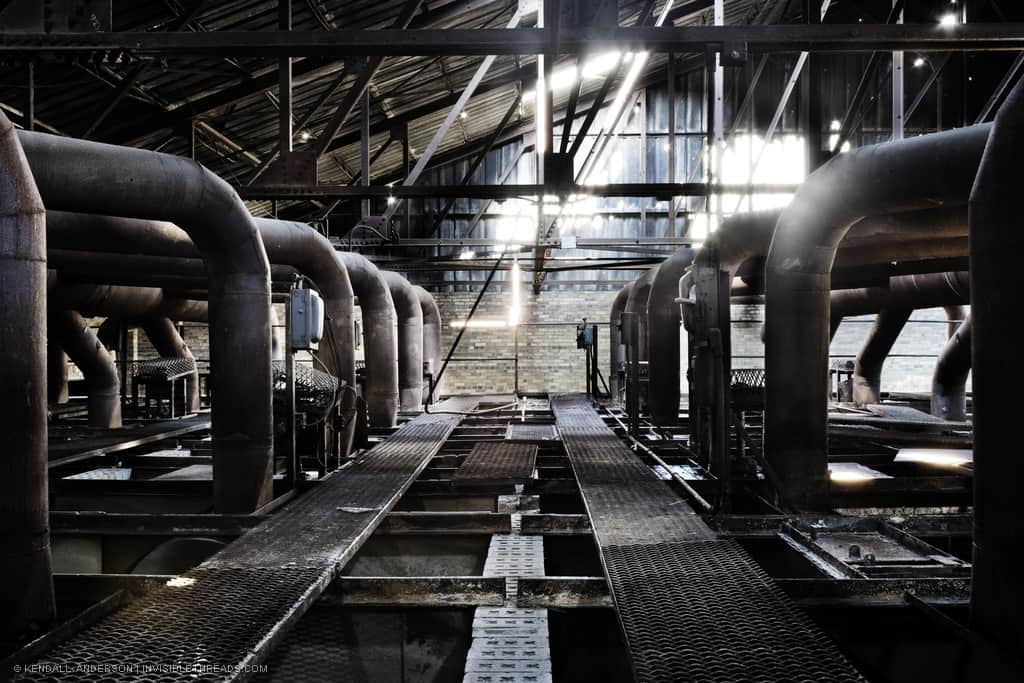
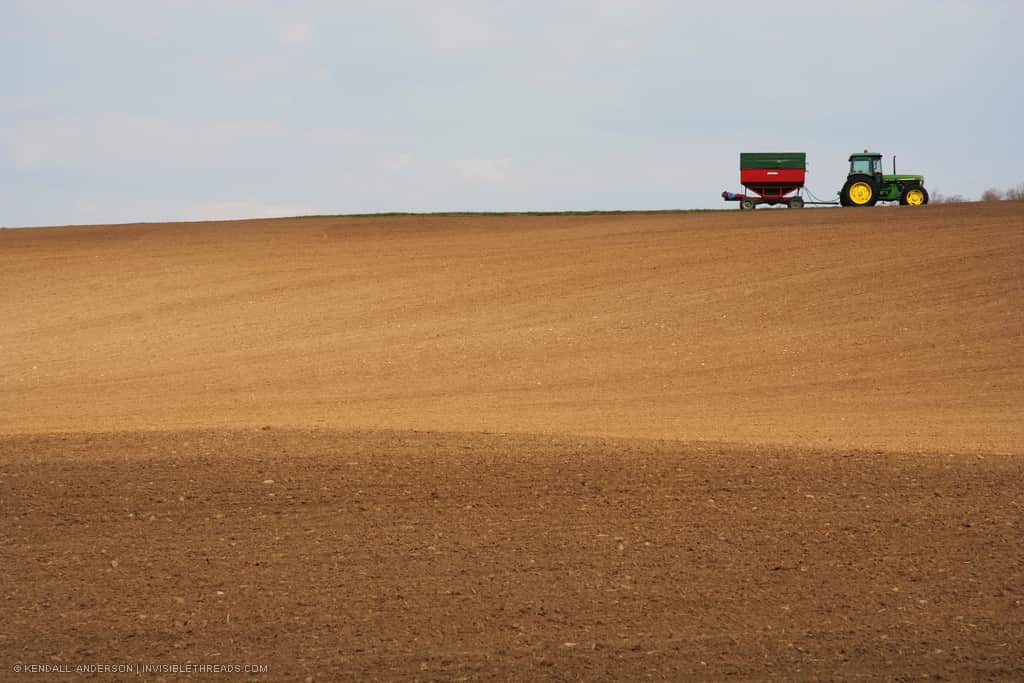
Example source
<it-carousel
pagination
pagination-label="">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 4c: Use custom labels inside pagination buttons
Use the pagination-label
attribute to provide a string for the labels inside the pagination buttons. Any instance of {{ index }}
will be replaced with the current slide number.
E.g., On the second slide, "Item {{ index }}"
would be changed to "Item 2"
.
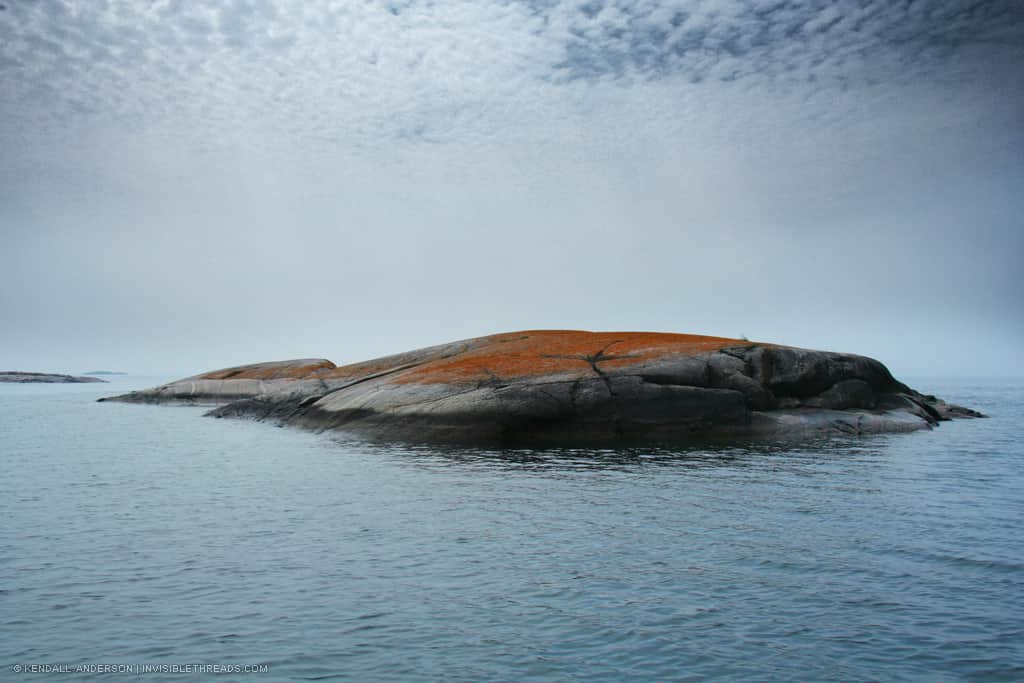
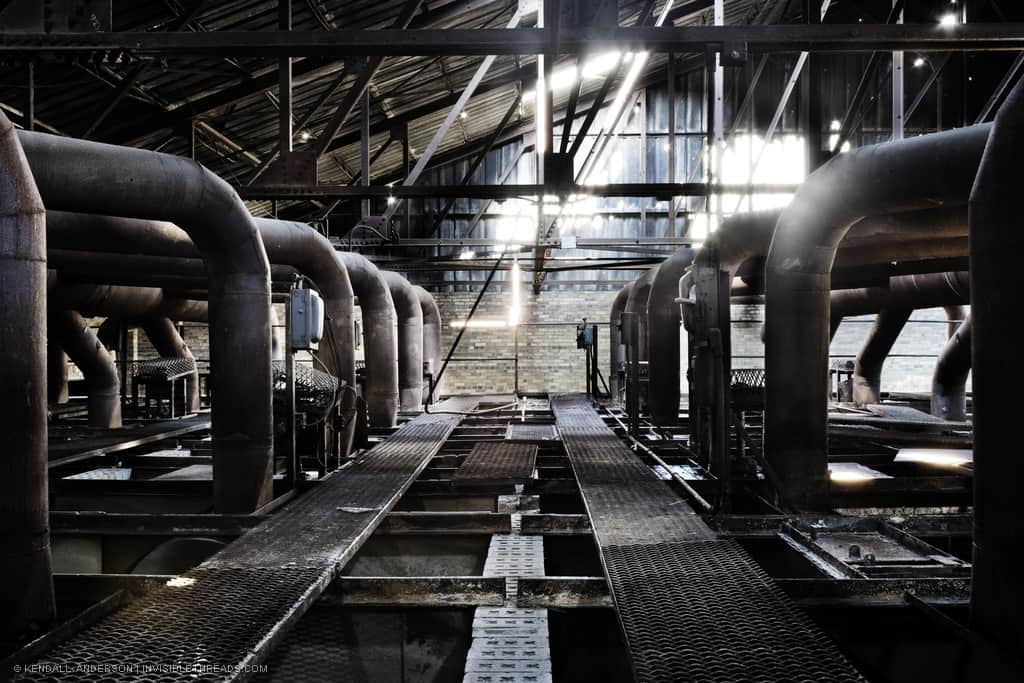
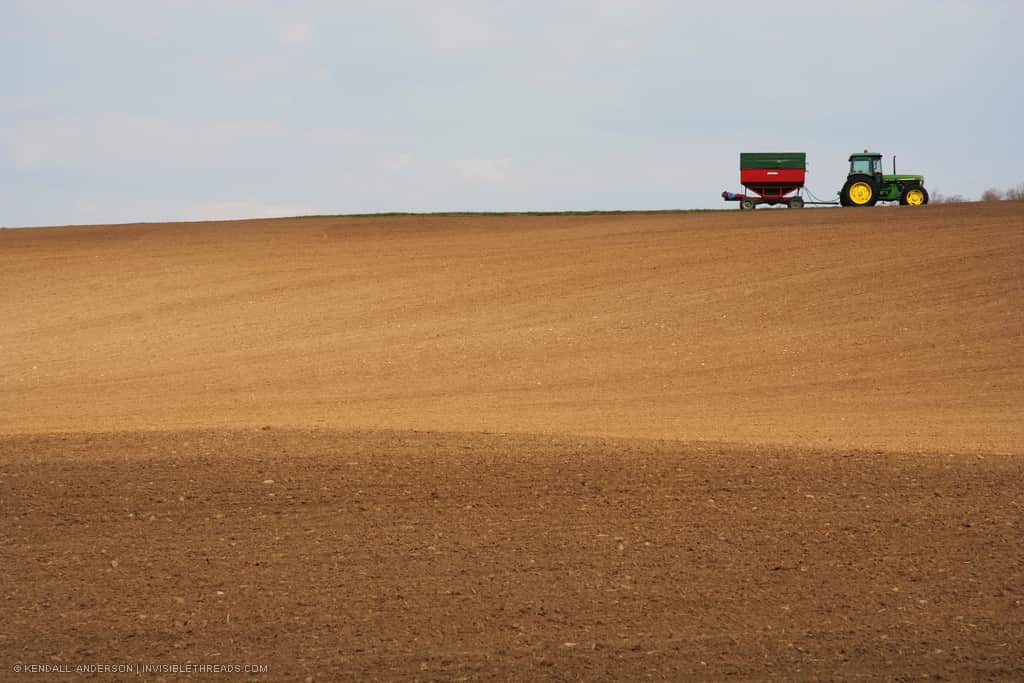
Example source
<it-carousel
pagination
pagination-label="Item {{ index }}">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 4d: Provide a unique label for each pagination button
Use the pagination-values
attribute to provide a string for each pagination button. This is a tilde-delimited list. If there are fewer values than slides, the label will use the default.
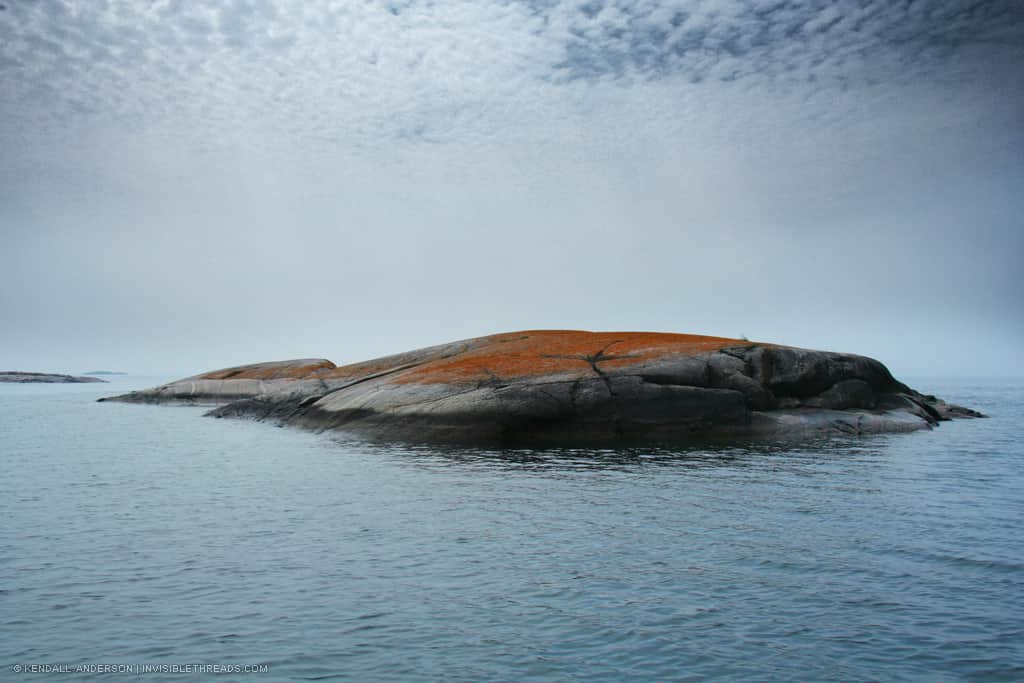
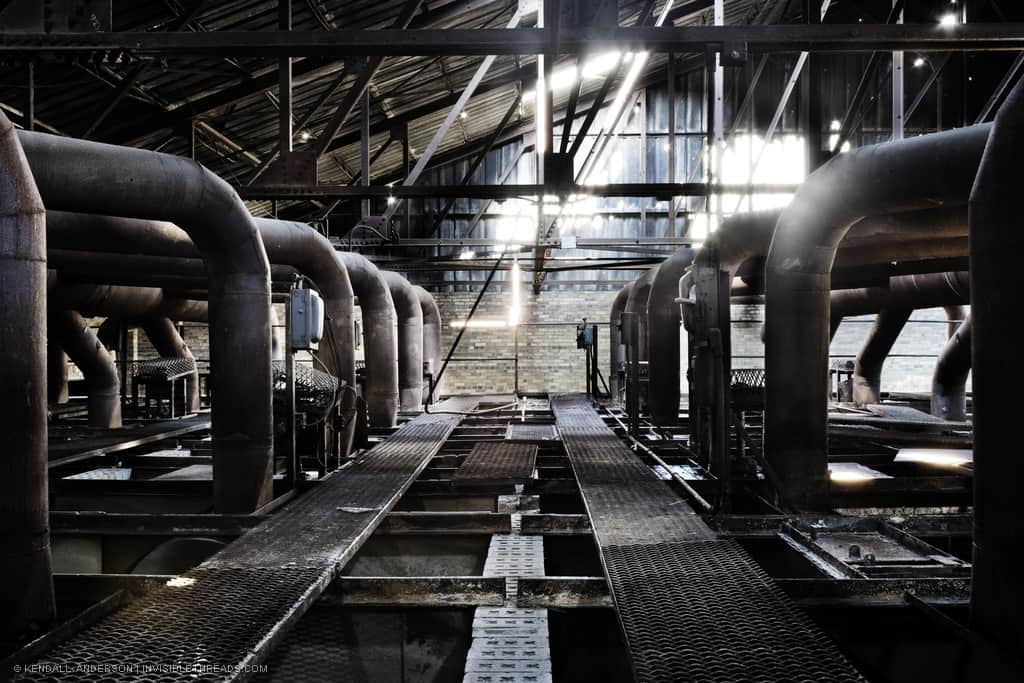
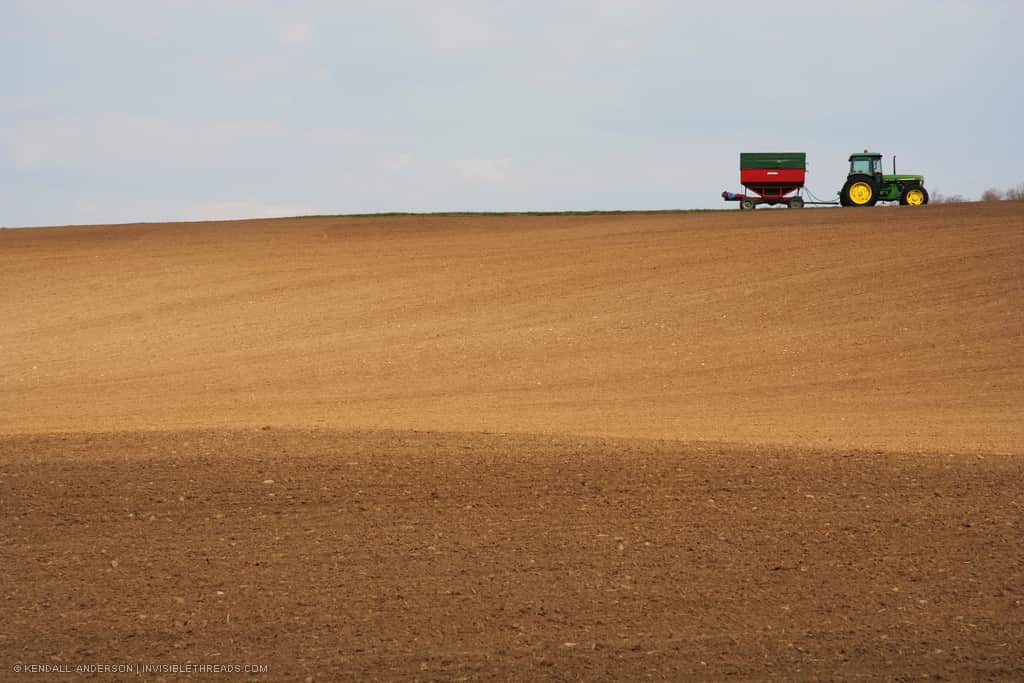
Example source
<it-carousel
pagination
pagination-values="Island~Pipes~Tractor">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 5a: Custom CSS styling
Use the part
attribute to target specific elements inside the carousel.
By default, the following parts are available for styling:
container
slot
button
,button previous
,button next
container-pagination
pagination-item
,pagination-item active
pagination-button
,pagination-button active
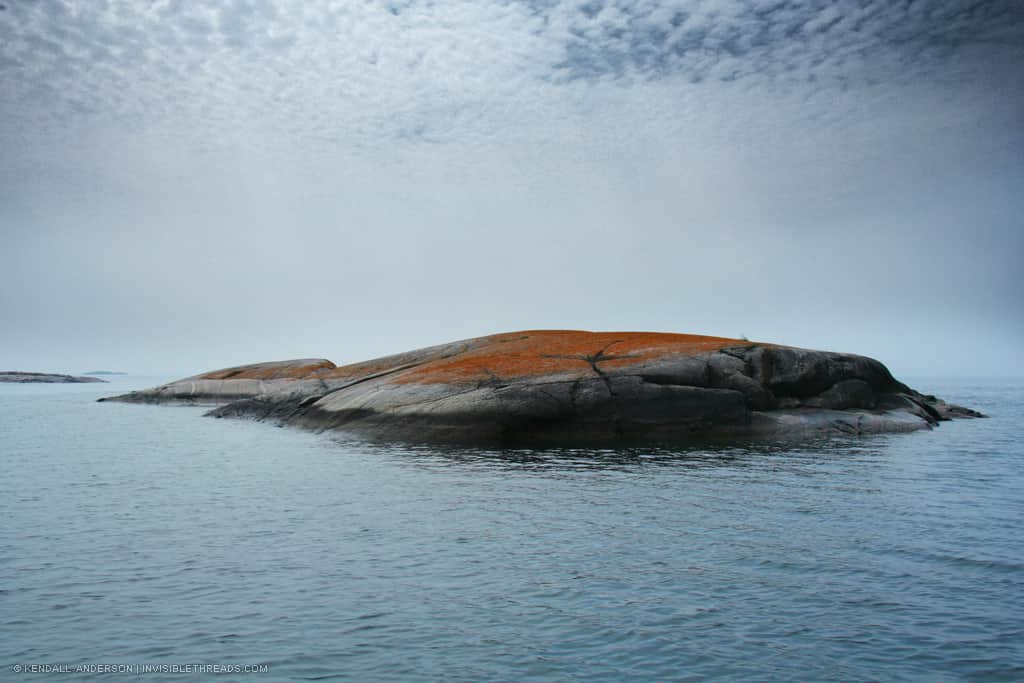
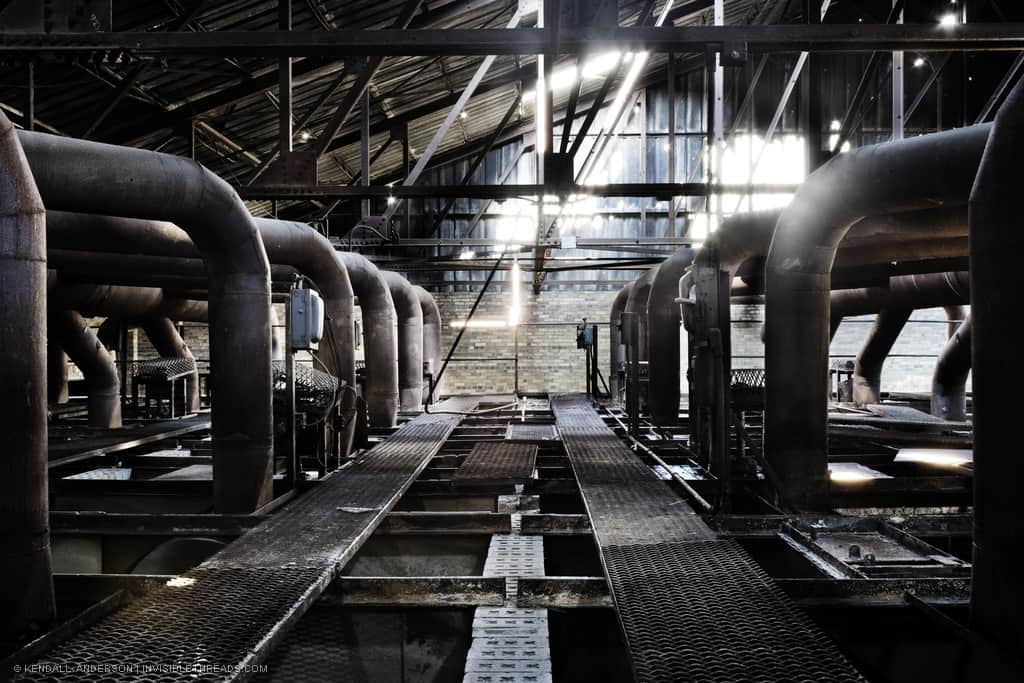
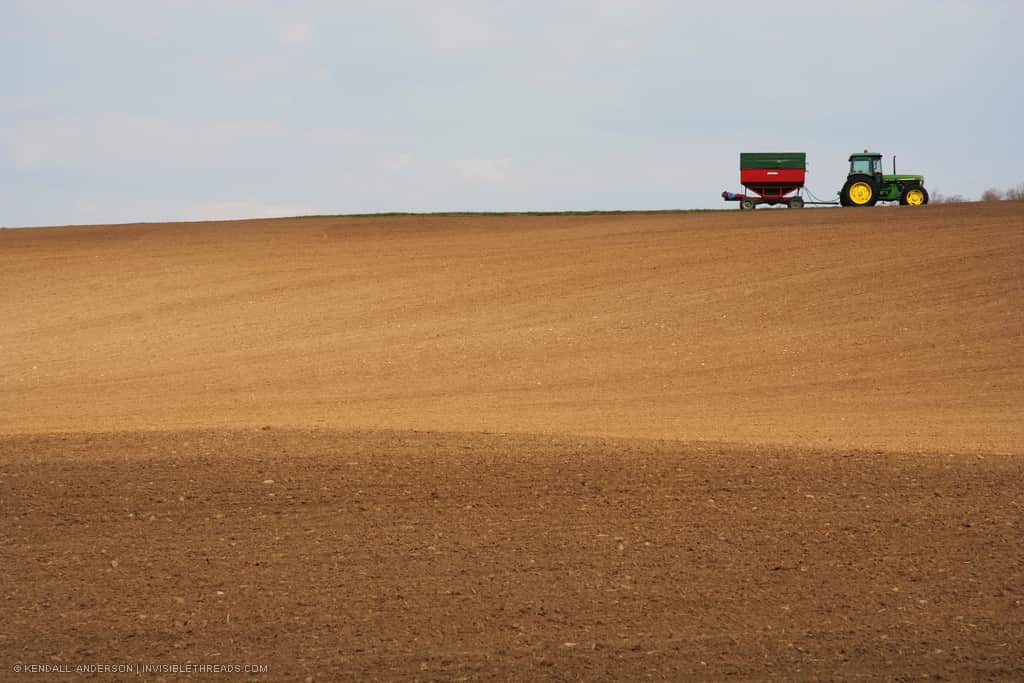
Example source
<it-carousel
id="example5a">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
#example5a::part(button) {
border: 5px solid blue;
border-radius: 1em;
}
#example5a::part(button previous) {
background: yellow;
}
#example5a::part(button next) {
background: lightblue;
}
#example5a::part(container-pagination) {
background: #ccc;
padding: .5em;
}
#example5a::part(pagination-button active) {
background: red;
color: white;
font-weight: bold;
}
Example 5b: No default CSS styling
Add the unstyled
attribute to create a carousel with no default CSS styles.
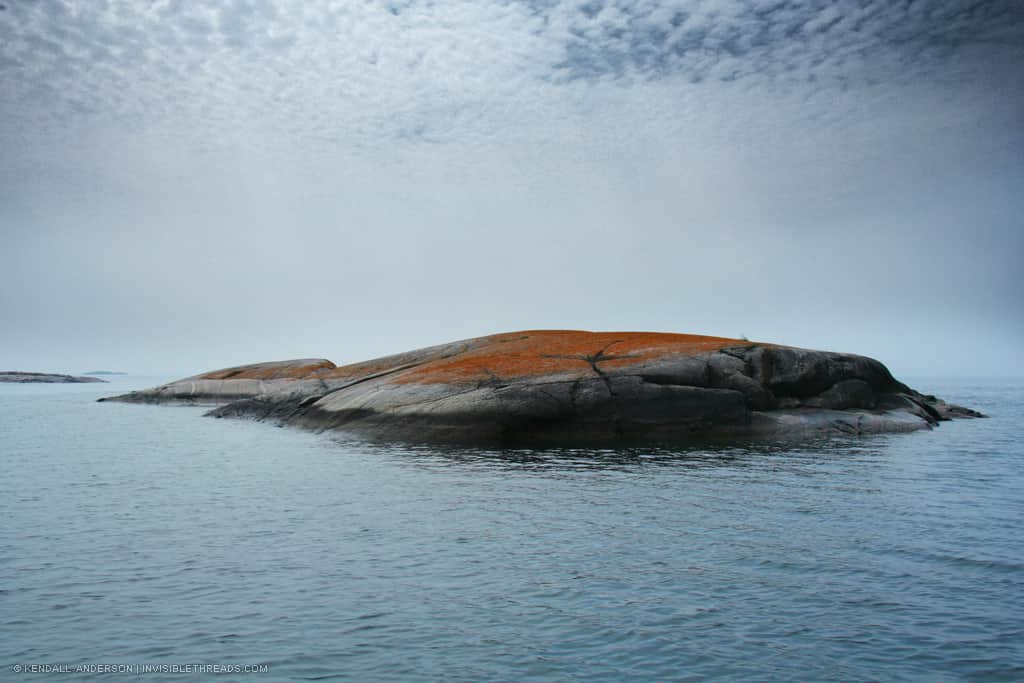
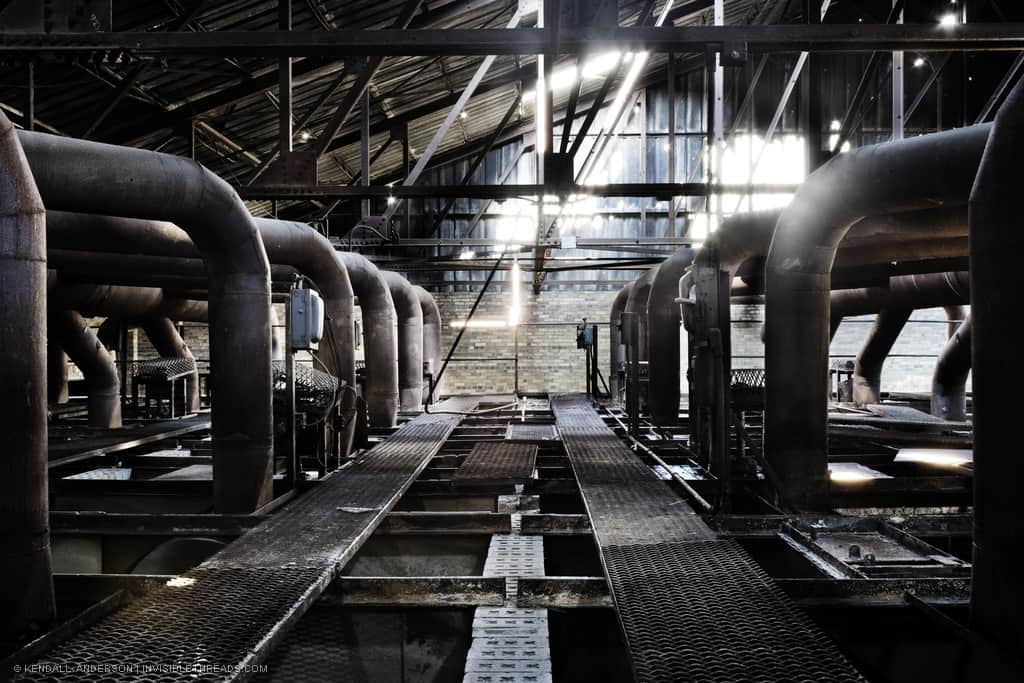
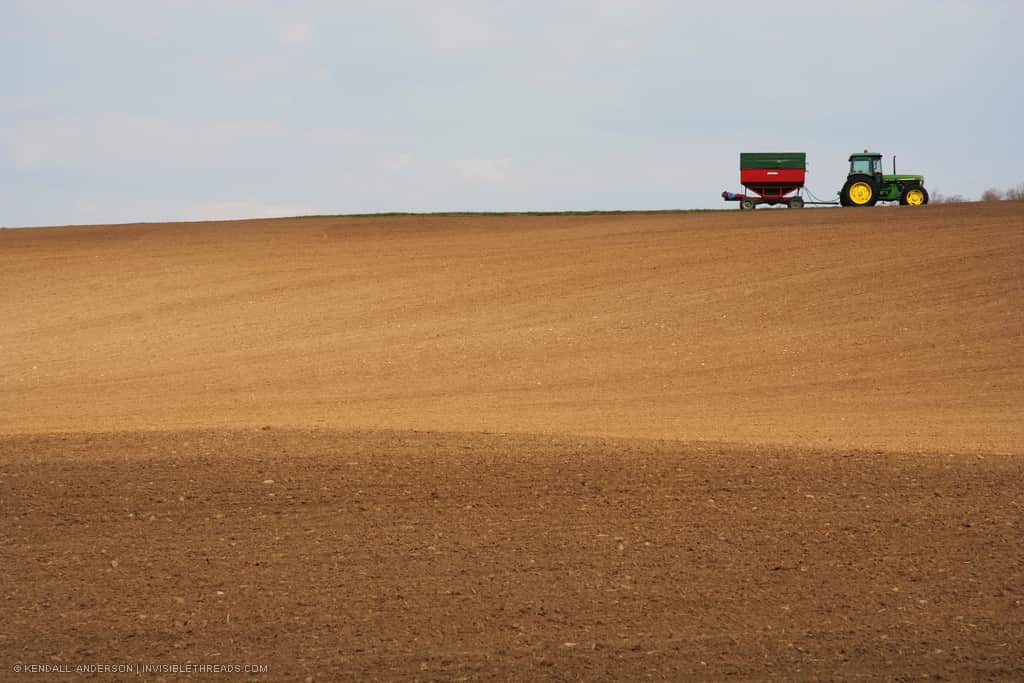
Example source
<it-carousel
unstyled id="example5b">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
Example 6: Custom HTML template
Maybe you need to make other changes to the default structure of a carousel that can't be accomodated with CSS styling alone. For that, you can provide your own HTML template. It might be overkill, and you'll need to ensure certain elements are present, but it gives you full control.
The (element).previous()
method will be attached to an element with id="btnPrev"
.
The (element).next()
method will be attached to an element with id="btnNext"
.
If you don't provide the <slot></slot>
element, the carousel will not have any slides.
You'll be in charge of what can be styled by adding part
attributes.
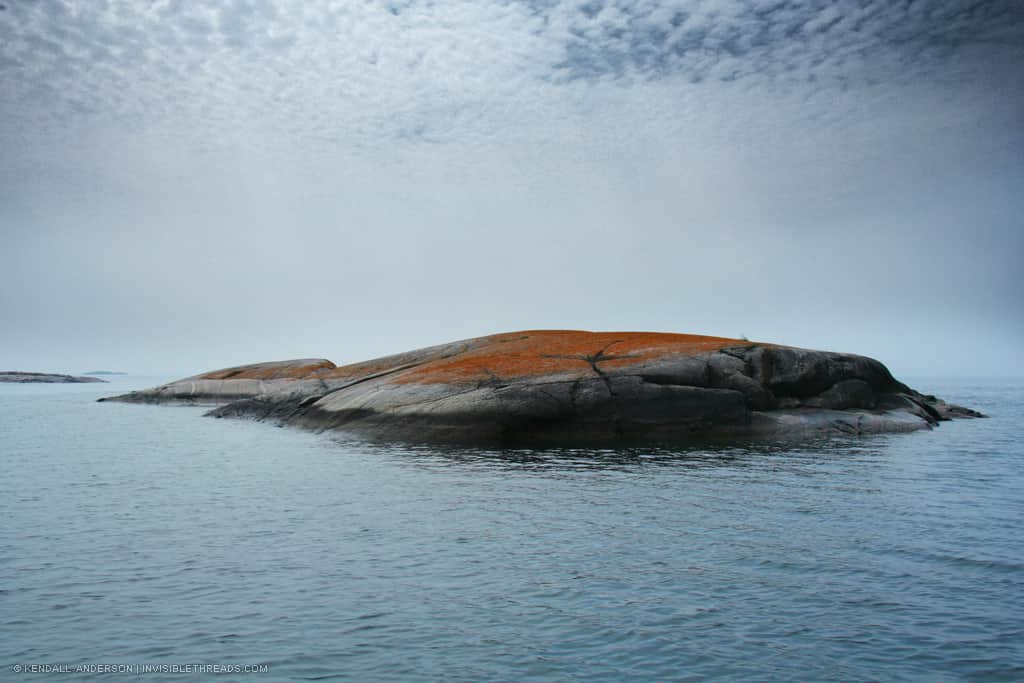
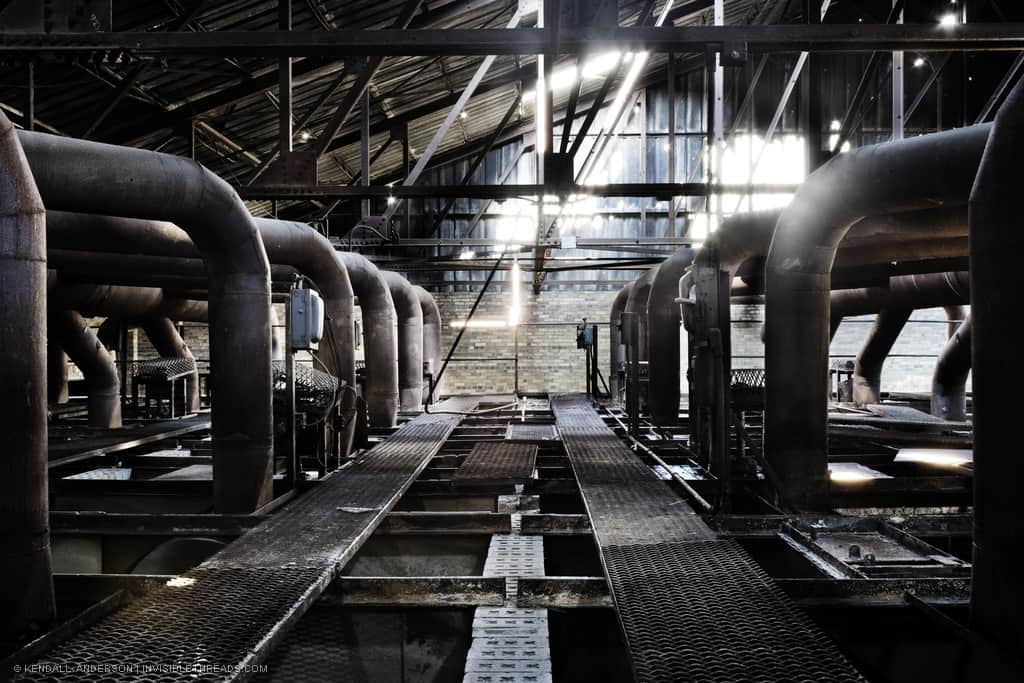
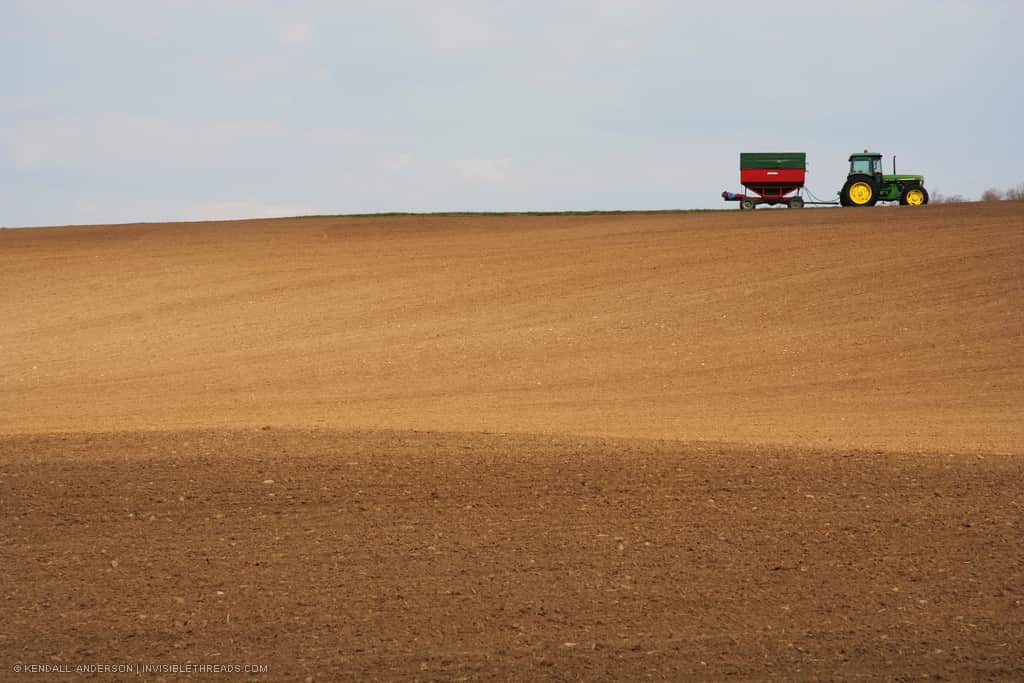
Example source
<template id="example-6-custom-template">
<div part="container">
<div part="buttons">
<button id="btnPrev" part="button previous">«</button>
<button id="btnNext" part="button next">»</button>
</div>
<div part="slot"><slot></slot></div>
</div>
<ul id="pagination" part="pagination"></ul>
</template>
<it-carousel
template-id="example-6-custom-template"
pagination
id="example6">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
#example6::part(container) {
position: relative;
}
#example6::part(buttons) {
position: absolute;
width: 100%;
top: 42%;
transform: translateY(-50%);
}
#example6::part(button previous) {
position: absolute;
left: -1rem;
}
#example6::part(button next) {
position: absolute;
right: -1rem;
}
#example6::part(button) {
font-size: 3rem;
font-weight: bold;
border: 0;
border-radius: 2rem;
height: 4rem;
width: 4rem;
color: white;
background: blue;
padding: 0 0 1rem;
line-height: 3.5rem;
box-shadow: 0 5px 5px rgba(255,255,255,0.5);
}
#example6::part(button):hover {
cursor: pointer;
}
#example6::part(pagination) {
list-style-type: none;
margin: 0;
padding: 0;
text-align: center;
}
#example6::part(pagination-item) {
display: inline-block;
}
#example6::part(pagination-button) {
border-radius: .75em;
border: 1px solid #ccc;
margin: 0 .1em;
}
#example6::part(pagination-button) {
background: ButtonFace;
color: inherit;
}
#example6::part(pagination-button active) {
background: black;
color: white;
}
Example 7: Controlling the Carousel via Javascript
Get the <it-carousel> element, and call its next()
and previous()
methods.
When called, these methods will also return a state object identifying the current slide index and total number of slides.
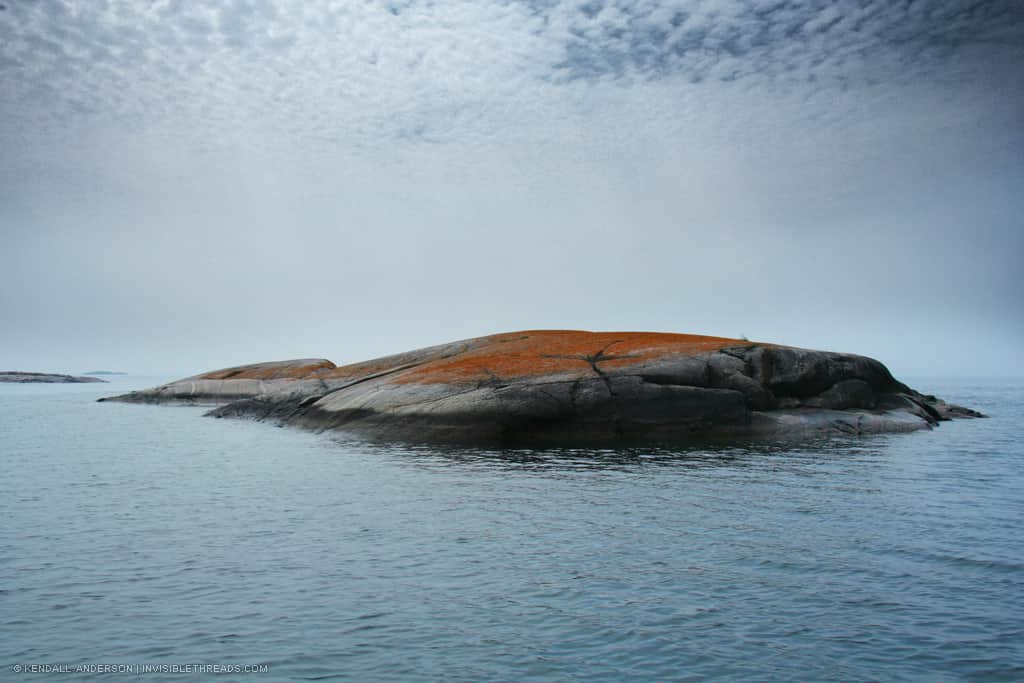
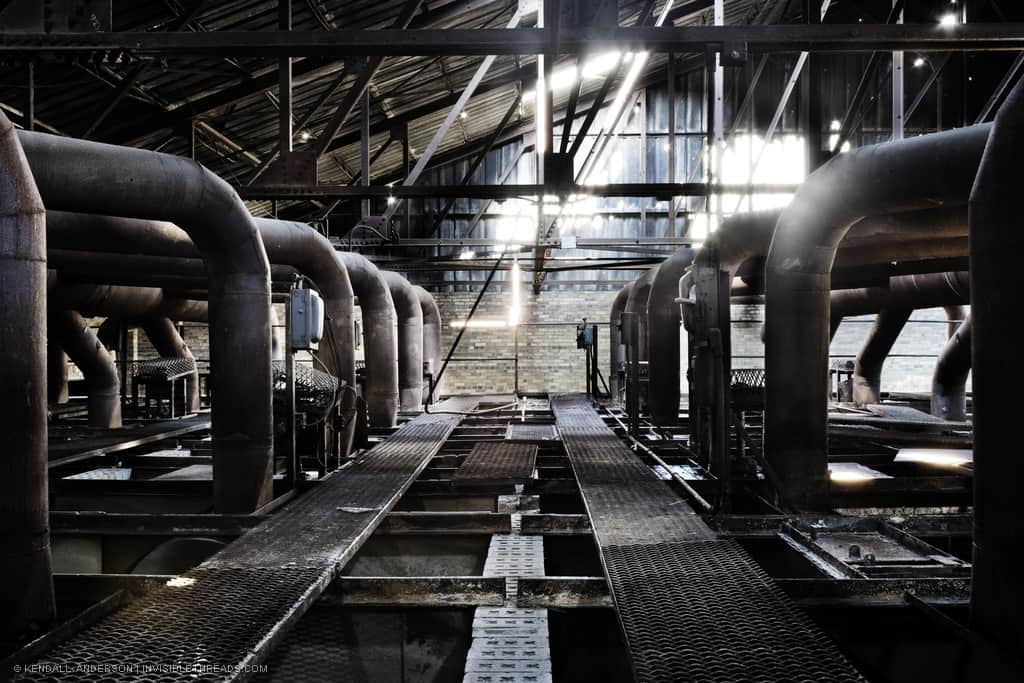
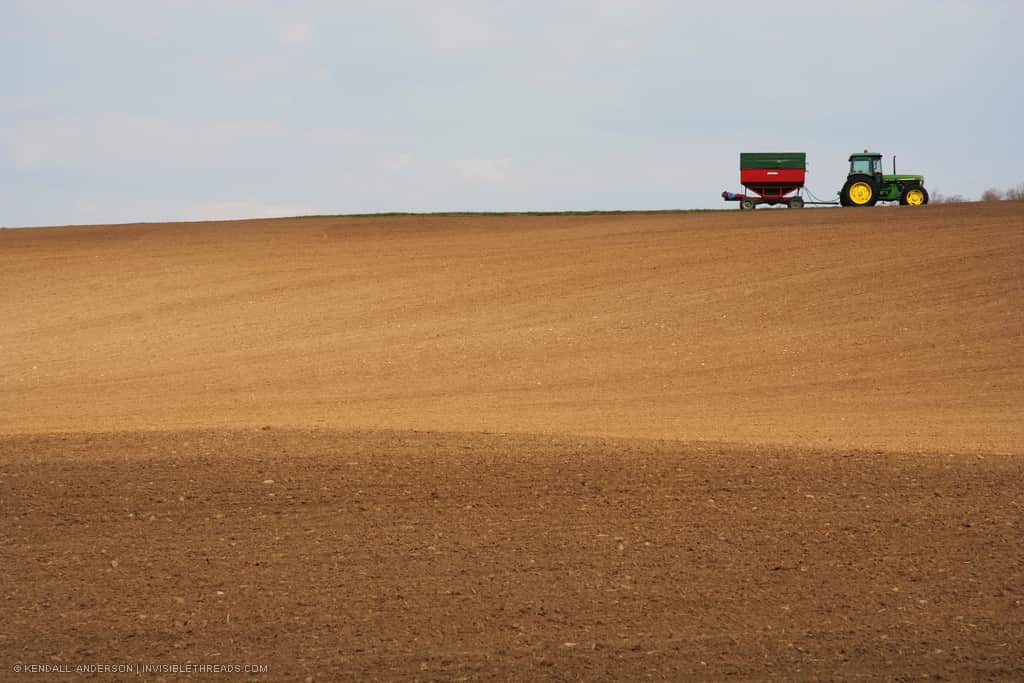
Example source
<it-carousel
id="example7a">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
<button onclick="document.getElementById('example7a').previous()">JS Go back</button>
<br>
<button onclick="document.getElementById('example7a').next()">JS Go forward</button>
Example 8: Detecting state changes with Javascript
How do I do something whenever a carousel changes? Create a Mutation Observer and attach it to the carousel.
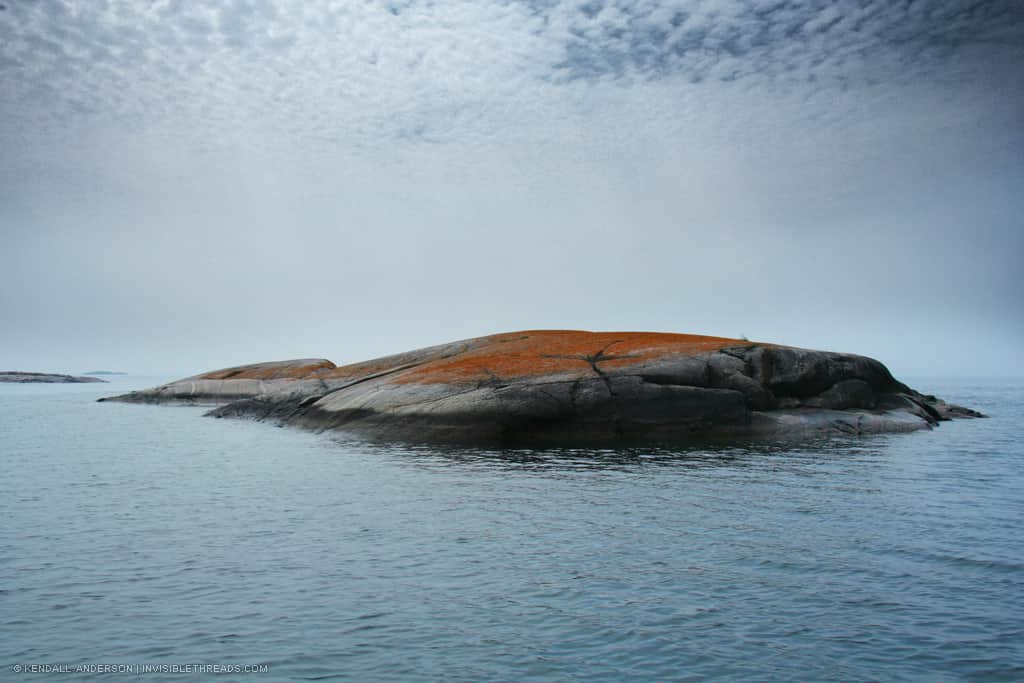
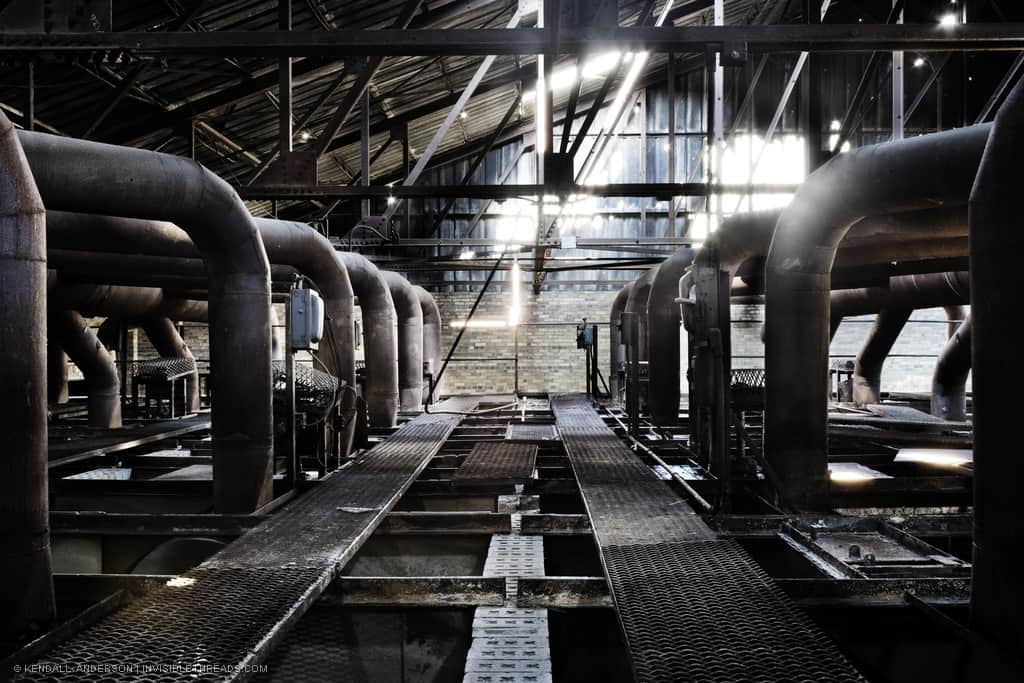
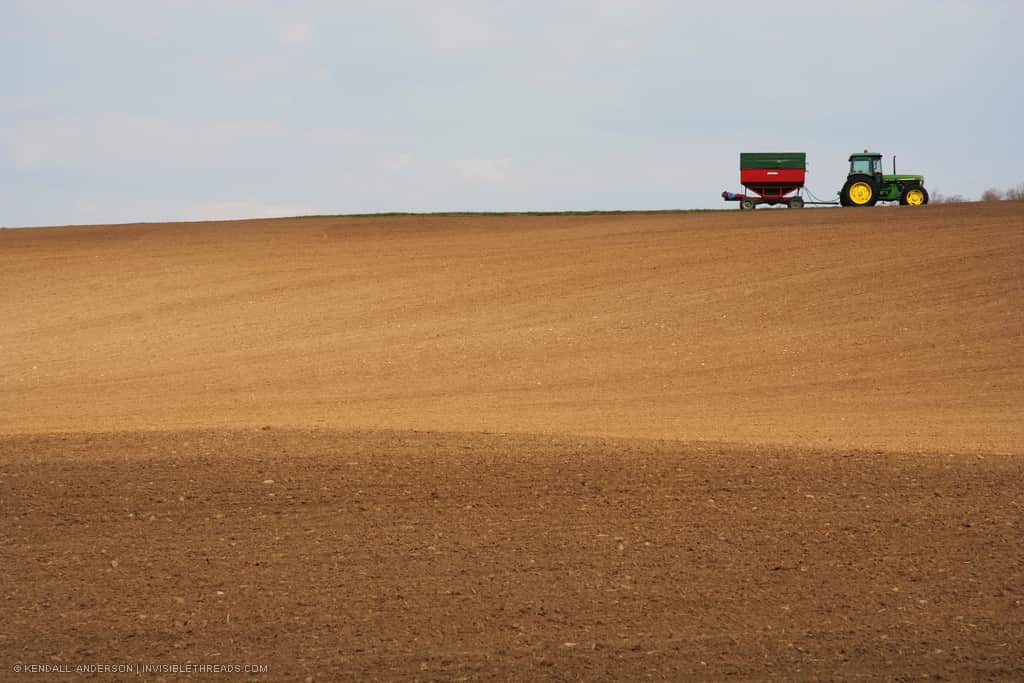
Carousel updated at timestamp:
Example source
<it-carousel
id="example8">
<img src="img1.jpg">
<img src="img2.jpg">
<img src="img3.jpg">
</it-carousel>
<p>Carousel updated at timestamp:
<span id="example8-timestamp"
style="border:1px solid #ccc; padding: .25em;margin-left: .5em;"></span>
</p>
const el = document.getElementById("example8");
const observer = new MutationObserver(function(changedItems) {
const elTimestamp = document.getElementById("example8-timestamp");
elTimestamp.innerHTML = Date.now();
});
const observerConfig = { attributes: true, childList: true, subtree: true };
observer.observe(el, observerConfig);